[This is mostly a technical exploration of Unity and my learning thereof. If you want to skip the technical stuff, go down to "final thoughts"]
Board/Game Controller
This project is still ongoing for me. They explained static variables and their use (slightly) and used a static variable to make sure only one instance of the gameManager is running at any time. Furthermore, they implemented DontDestroyOnLoad(gameObject) to make sure that the gameManager is not destroyed between scenes, since it will have to keep track of score/health/levels throughout the game. A persistant instance of gameManager must always be running. This gameManager GameObject is saved as a prefab and the Main Camera has a loader script, which Instantiates the GameManager prefab if instance==null. It’s a basic way of making sure your Game Manager is consistent and persistent with pre-selected public variables.
Moving Objects
Abstract classes are used. I know how to use abstract classes and how they work in Java, but I’ve never had cause to write one myself, so I guess I should pay attention. In C#, methods in abstract classes are written with virtual (e.g. protected virtual void Start()), which allows them to be overridden (by e.g. protected override void and declaring a class as public class Name : MovingObject) . Also, I just found out multiplication is more efficient than division, so if multiple divisions are made with a variable, it is more efficient to convert variable to inverse and do multiple multiplications with this inverse. How clever. And all the programmers with degrees are saying “duh” at me right now.
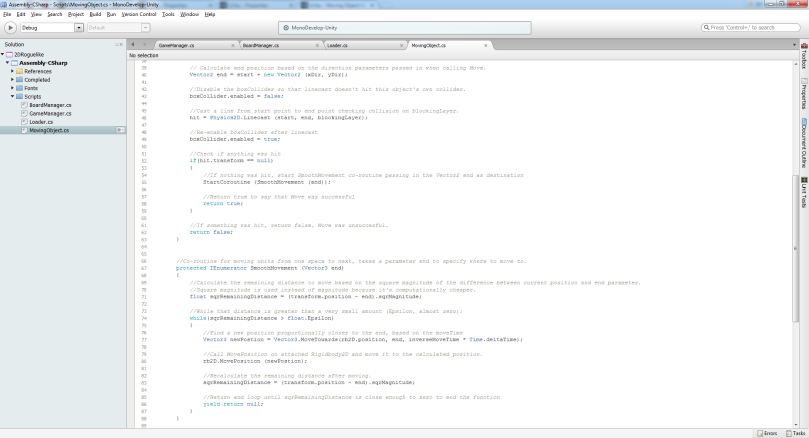
Anyway, we’re using Abstract Classes here because both the enemy and the player will be using the Abstract MoveObject class, passing either a Player or Enemy component to the class. I highly recommend both checking the YouTube comments (the creator comments there) and the script on the unity page, as it has a comment on every line, as you can see in the image above.
I got a compile error from the Wall script, even if I copy-pasted his code, so I just used the Wall script included in the assets. I’m light on myself now but will figure stuff out once I start on my own engine, which should be by next week. I’m not sure how Unity’s Script Editor (MonoDevelop) works with sorting out compile issues. Usually I don’t realize there’s a compile error at all until I try to use the script from the Unity GUI. Will look into that.
—
Ah. I just needed to press F7 (build project). And it showed me that the errors happened because the assets already contained scripts and classes with the given names. So uh. I’ll just use their scripts and work along with the GUI stuff. Freaken things sucks. We’ll do it live!
Player/Enemy Animator Controller

This is quite nice. You set up animation states and determine when/how the transitions occur. I’m not focusing on animation yet, but will need to get back to this once I’ve set up the basic game logic and stuff.
Player/Enemy Script
They introduced [HideInInspector] which hides public variables from unity, but makes them accessible to scripts (if public). They also introduced Unity’s built in OnDisable function (self explanatory), and demonstrated how to inheret and override an abstract class and its methods. Furthermore, to load a new (or reload a current) scene, they used Application.LoadLevel(Application.loadedLevel); To assign a GameObject not specified, the used target = GameObject.FindGameObjectWithTag(“Player”).transform;
The important thing to note here is that the Player script has one new instance running per level, so any variables (such as health/food) that need to be carried over to the next level, must be stored in the GameManager when the Player/Enemy/Whatever script is OnDisabled, since the GameManager is always running. I’m assuming that this is good practice for Unity.
This guy has an interesting way of doing things. He sets the value of food in the player script. Then he sets the damage that the player does in the enemy script. I would rather have set the damage and all that in the player script, and called functions from the enemy by passing ints from the player. public void attack(int damage){}; Not sure whether my method would be better at all though.
Also to note is that he likes writing “return” in the middle of a method. I’ve been told that’s bad practice because it’s difficult to see all the exit points in your method if you do that. I agree with that assessment, so I’m going to continue using ifs en elses to reduce the exit points to a small number.
UI + Audio
More or less the same as the previous tutorials. A canvas gameObject is used with images on top that are anchored to the corner, and so on. The script then updates the text every time there is a change from damage or item pickup or level change. Simple stuff.
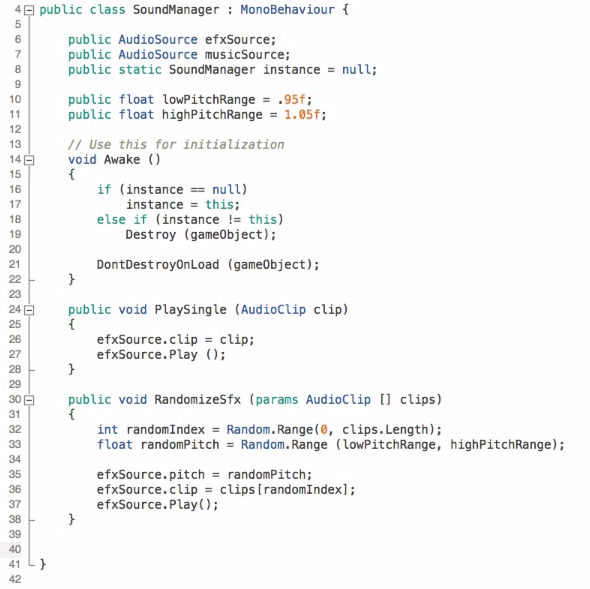
For sound, they use the SoundManager Game Object, with script SoundManager.cs. A cool between this and the previous sound manager is that they set public floats lowPitchRange and highPitchRange to subtly change the noises. Clever method I’d like to employ for non-dialogue and non-music sounds.Furthermore, they declare public void PlaySingle(AudioClip clip){}, and public void RandomizeSfx (params AudioClip [] clips){}. I thought that was pretty simple and straightforward. I’d probably use the exact same method, though with dialogue added as a third Audio Source.
Also: Blocking Layer
So, I didn’t really understand the “Blocking Layer” tickbox in Unity, so I checked up on that. It turns out (from Unity’s page on Blocking Layer) that basically, you can set any 2D or 3D object to a certain layer. You can then set which layers interact with which layers. For example, you can have enemies all from EnemyLayer. So the player can not walk through an enemy, but the enemies can walk through each other, for example. I also suggest looking at Unity’s cool Vector Cookbook page. It’s awesome for vector computations.
Final Thoughts
I am now done with Unity tutorials and will be working off of the documentation and Stack Overflow and whatnot from this point on. With this I am “ready” to begin with the game. Not actually ready, not really ready, not at all ready, to be honest. I need to know Unity, C#, and programming in general a lot better, before I can do this properly.
But fuck that, I don’t care. It’s time to start and see what I can achieve. I’m not going to increase my XP without grinding on a project like this.
I noticed from the comments that there are suddenly a LOT fewer people responding or interested in the intermediate tutorial. This, coupled with people from the beginner tutorial complaining that the “scripting part could not be less intuitive” and that “I like everything except that stupid scripting part” gives me the impression that there are actually a lot fewer competent+driven people out there willing to make games than I feared at first. I mean, with the amount of zero-effort games out there (see The Asset Flip by Jim Sterling), I think we actually stand a chance to release something that I’ll at least be willing to put put our for free, if not release for money.
Oh, and Zane and I decided on a studio name for now: